Spring Boot Standalone and Clustered Java Applications with BitssCloud
Since the very foundation, BitssCloud PaaS evangelizes the idea of making an application deployment process as easy and universal as possible. Keep following our mission, today we are glad to introduce another newcomer within a family of certified Java-powered stacks – Spring Boot server for standalone and clustered applications or microservices.
With BitssCloud Spring Boot stack template, you can effortlessly run various Java-based applications inside the cloud via easy-to-use graphic interface or automation scripts. The following Java project types can be hosted within this server:
- JAR – for standalone Java apps or stateless microservice instances, created using Spring Boot, Dropwizard or Spark frameworks
- WAR – for web applications with the embedded servlet container
In this way, Spring Boot enables you to keep things portable, whilst BitssCloud ensures quick delivery of applications to production and their comprehensive management via GUI, API, or Cloud Scripting.
Creation of Spring Boot Environment
So, log in to your BitssCloud dashboard, and let’s get started.
1. First of all, you need to create a New Environment – use the same-named button to launch the topology wizard. Switch to Java language tab and choose the SpringBoot template within the application server layer at the left-hand panel as shown below. Set the number of allocated resources, type your environment name, and click Create to proceed.
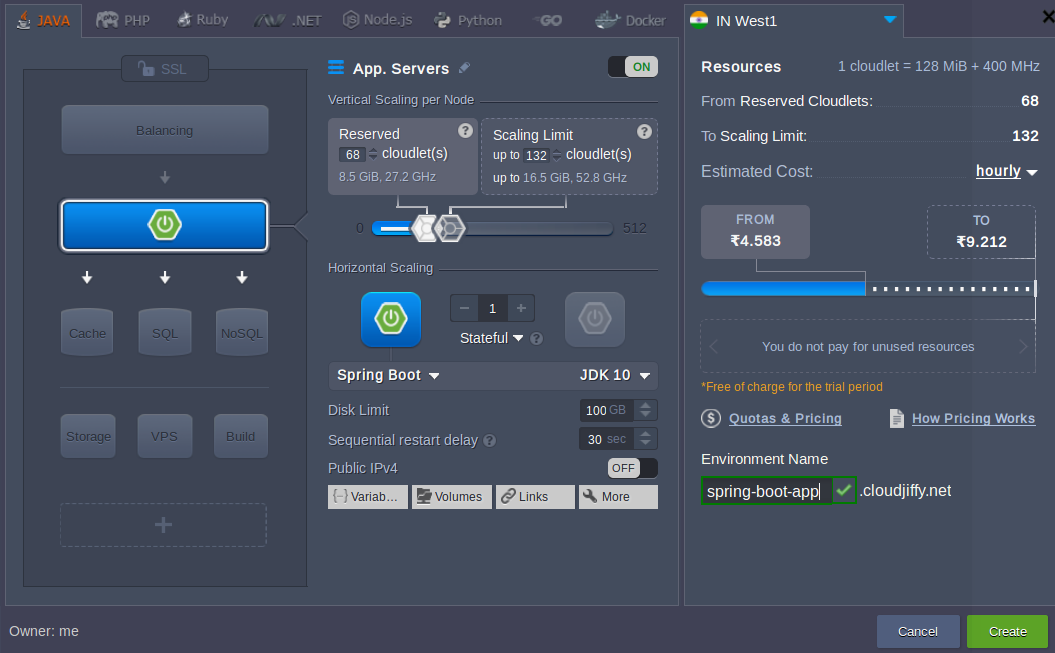
Versions Compatibility:
- The indication with 1.x-2.x label implies that the BitssCloud-managed Spring Boot server can run any application, built upon these versions
- The current custom Spring Boot stack is provisioned within BitssCloud Platforms of 4.10 version and higher.
2. When your new environment appears on the dashboard, you can click Open in the browser to launch the pre-installed Hello World application sample.
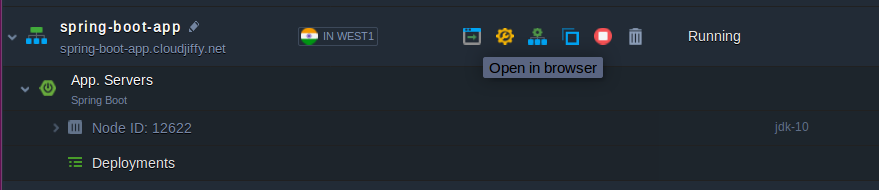
In this way, you can make sure your Spring Boot instance is up and running.
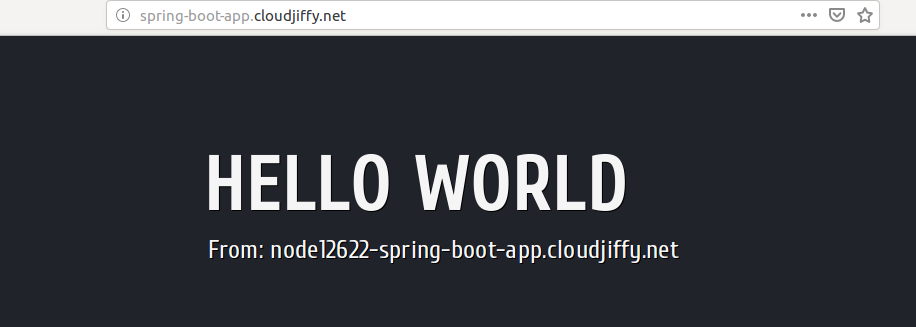
Now let’s consider how to integrate your custom Java application into the created Spring Boot environment.
Signup Now for a 14-day free trial to try out Springboot on BitssCloud.
Deploying Java Applications to Spring Boot
To launch your Spring Boot project inside BitssCloud, you need to preliminary pack it into an executable archive with all the appropriate compiled classes and associated resources inside (including an embedded servlet container for handling self-contained applications). The following archive types are supported:
- JAR – the most common Java-archive type; should either contain a manifest with declared entry point class or be built as all-in “fat” JAR or nested JAR file
- WAR – for the deployment of applications with embedded servlet container; in particular, should be used for JSP-based projects for solving known supportability issues within Spring Boot
ZIP Bundle
By default, any executable JAR file, created by Spring Boot, contains property files and additional settings. However, in the case of production deployment, it is more convenient to distribute these files outside of the packaged archive. For example, this can be applied to externalized configuration property files (application.property, application.yml) or logging configurations (log4j2.properties, logback.xml).
To simplify application deployment in these cases, BitssCloud supports the deployment of a ZIP bundle, which may contain an executable JAR file and any additional files or directories.
While unpacking a ZIP bundle, BitssCloud processes all the included folders to detect the runnable JAR. To be considered as an execution point, a JAR file should contain the Main-Class declaration inside its META-INF/MANIFEST.MF manifest file. Once such JAR file is located, BitssCloud will run JVM with “java –jar /path/to/jar” parameters inside the directory that corresponds to the root folder of the deployed archive.
Example
Below is a sample directory structure of a ZIP bundle that can be used for deployment into BitssCloud .application.zip
|
+-config
| +-application.properties
| +-log4j.properties
+-lib
| +-my-springboot-app.jar
+-some_directory
| +-additional_file1
| +-additional_file2
+-additional_configuration.yml
If deploying such an archive, BitssCloud will run JVM with the “java -jar lib/my-springboot-app.jar” arguments from a directory that corresponds to the root folder of the unpacked application.zip archive.
Application Deployment
BitssCloud provides versatile possibilities for your application deployment into the Cloud, allowing to choose the most preferable one.
- Manual Deployment
The most evident and comfortable approach is to perform this manually through the convenient BitssCloud GUI.As an example, give a try to a sample Spring Boot project, which represents a simple application to store the messages you create – you can either build it from the sources or deploy the already built JAR.
For that, Upload the archive with your app via Deployment Manager and initiate its deployment by selecting the destination environment (the previously created spring-boot-app in our case).
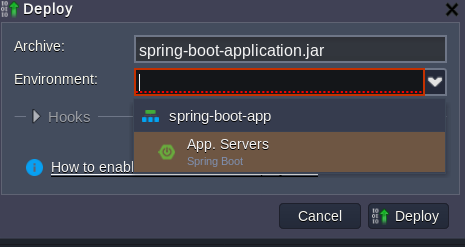
In the opened dialog, confirm Deploy with the same-named button and wait till the corresponding task is finished.Note: Support of WAR archives deployment through dashboard’s Deployment Manager is provided starting from BitssCloud 5.0.6. For preceding platform versions, this operation can be accomplished via BitssCloud API or Cloud Scripting.
Checking Logs
When the deployment is finished, give the system some more time to run the required services (where delay directly depends on your project complexity) – the current progress of this operation can be tracked in real-time via the springboot.log server log.
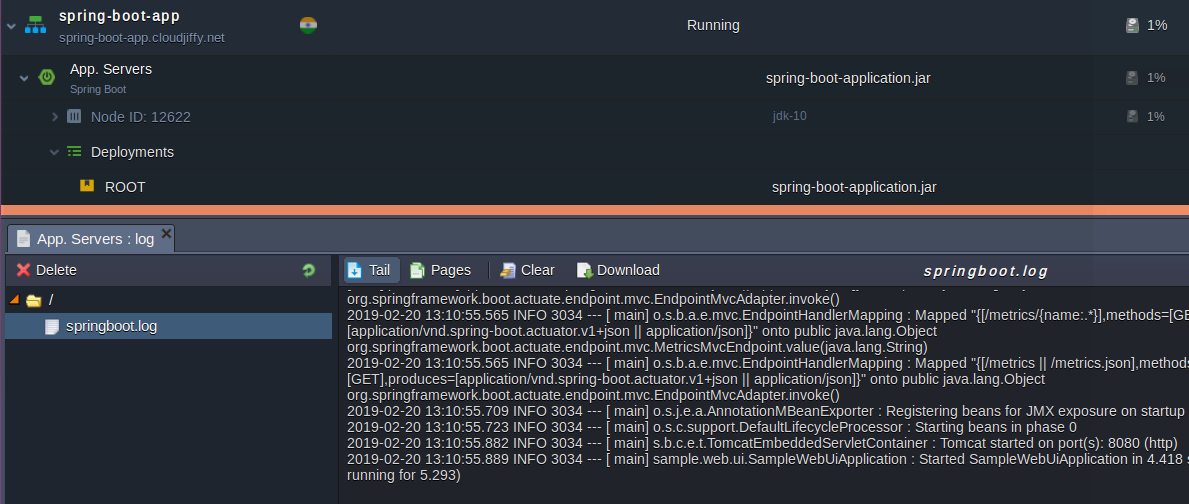
After completion, your application web interface (if such is run on the default: 8080 port) can be accessed identically you’ve done this for preinstalled Hello World – by clicking Open in the browser for your environment.
Ways to Build a Spring Boot Application
To create an appropriate archive file for your application hosting inside BitssCloud, either Gradle or Maven build tools can be used.
- The minimal base for the Gradle build script (build.gradle) is listed below, where parameters in curly braces should be substitutes with your custom values:
buildscript { ext { springBootVersion = '{X.X.X.RELEASE}' } repositories { mavenLocal() mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") } } apply plugin: 'java' apply plugin: 'eclipse' apply plugin: 'org.springframework.boot' sourceCompatibility = 1.8 targetCompatibility = 1.8 jar { baseName = '{your_app_name}' version = '{your_app_version}' } repositories { mavenLocal() mavenCentral() } dependencies { compile("org.springframework.boot:spring-boot-starter") } task wrapper(type: Wrapper) { gradleVersion = '{used_gradle_version}' }
To build an executable JAR file with this script, use the following command:
./gradlew clean assemble
The generated archive will be stored upon the ..build/libs/{app_name}-{app-version}.jar path.
- The minimal base for pom.xml Maven project description includes the following parameters (where parameters in curly braces should be substitutes with your custom values):
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>{Х.Х.Х.RELEASE}</version> </parent> <groupId>org.springframework</groupId> <artifactId>{your_app_name}</artifactId> <version>{your_app_version}</version> <properties> <java.version>1.8</java.version> </properties> <dependencies> <!-- Compile --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
To produce an executable JAR with Maven, execute the following command:
mvn clean package
Your app archive will be placed at the …target/{app_name}-{app-version}.jar location
BitssCloud Maven Plugin
As a more convenient joint build & deployment option, consider the BitssCloud Maven plugin, which is aimed to facilitate your application delivery to the Cloud. Integrate it into your Maven project’s pom.xml configuration file and get the ability to build an application archive and instantly push it to a target environment with a single command.
CI/CD Tools for Java Applications in the Cloud
Dedicated Maven Build Node in the Cloud
In case you do prefer working via GUI, leverage the dedicated Maven build node the BitssCloud Platform provides out-of-box. Being included to an environment alongside with Java application server, it can be used to fetch, compile and deploy the application from its sources within a specified remote GIT/SVN repository.
CI/CD Automation Add-On
In addition to all the above-mentioned options, BitssCloud offers a special Git-Push-Deploy add-on for continuous application deployment from GitHub & GitLab repositories via the automatically integrated CI/CD pipeline. It can be especially useful in case your project is under intensive development yet, which implies lots of repetitive commits. With this solution, your application will be automatically rebuilt and delivered to a target server upon any change is made within its code, making the newest version available via the corresponding domain in a matter of minutes.
Maintaining Your Spring Boot Server
The majority of basic server management operations can be performed right via BitssCloud UI with the appropriate embedded tools, e.g.:
- use the built-in Configuration Manager to create or upload new files, edit or delete the existing ones, set up mount points and manage exported data from other servers
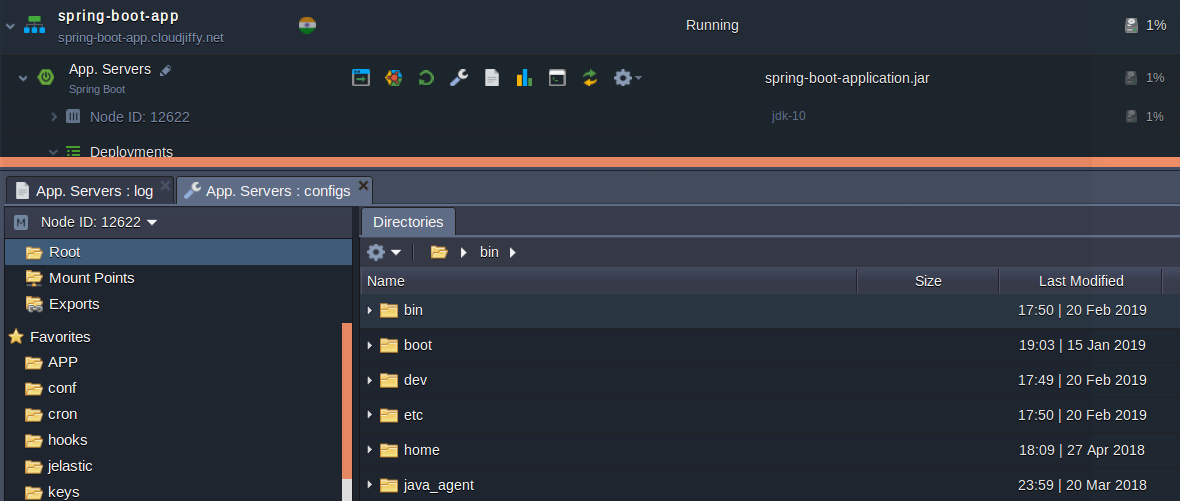
- set up custom Java options and arguments for your server by adjusting the JAVA_OPTS and JAVA_ARGS environment variables
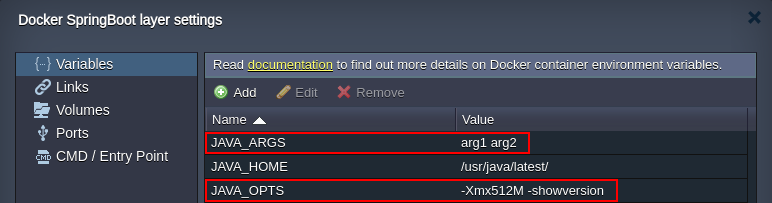
- explore server Logs to get the details on run operations for efficient service administration and troubleshooting
- track the Statistics on consumed resources to be aware of the capacities your server actually needs and define the best approach for their allocation
For more complex maintenance operations, the BitssCloud SSH Gate can be used. It allows managing your containers remotely with full root access provided. For that, you just need to connect to your account with the previously generated SSH key pair. And the following tips can come in handy when working with your Spring Boot server:
- your application files can be located in the /opt/shared/apps/APP directory, which is considered as “current” or “working” for Java process
- Java Virtual Machine (JVM) configuration parameters can be customized within the /opt/shared/conf/variables.conf file (e.g. to enable remote debugging or pass any additional arguments to JVM)
- to inspect log files, refer to the /opt/repo/logs directory
- the /opt/shared/home location is considered a home directory
- JDK is located in the /usr/java/default directory
- to restart your application, execute the sudo service cartridge restart command
In particular, the ability to operate servers via console can be especially useful when handling non-web Spring Boot applications.
Automatic Scaling for Spring Boot Server
BitssCloud Platform provides real-time elastic scalability that is available out-of-the-box for any server. By enabling both automatic vertical and horizontal scaling, you can make your Spring Boot application fully adaptable to changeable workloads.
Automatic Vertical Scaling
The automatic vertical scaling is enabled by default and ensures your service remains available during the load spikes, and at the same time, eliminates the need to pay for unused resources. Just decide on a maximum limit of resources (set with cloudlets) your application may consume and BitssCloud will automatically adjust the maximum memory size (-Xmx) for your application based on these capacities, for example:
- 8 cloudlets (1GiB RAM) set the maximum heap size to 819 MB
- 16 cloudlets (2GiB RAM) set the maximum heap size to 1638 MB
To customize -Xmx or any other JVM options, edit the /opt/shared/conf/variables.conf file either via Configuration Manager or BitssCloud SSH Gate.
Automatic Horizontal Scaling
The automatic horizontal scaling functionality allows adjusting the number of web and application servers according to the resources consumption of your application. It is implemented by means of the tunable monitoring triggers that are based on the usage of a particular resource type:
- CPU
- Memory (RAM)
- Network
- Disk I/O
- Disk IOPS
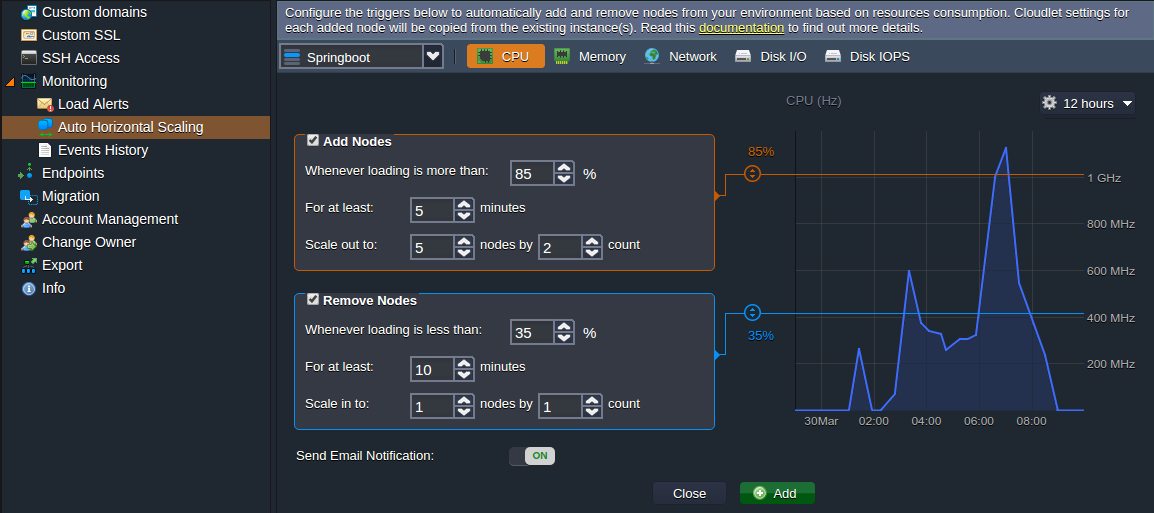
Note: When a server is scaled out (both with a trigger or manually), an NGINX load balancer will be automatically added to your environment, with pre-configurations for sticky session load balancing. Alternatively, you can switch the used balancer stack to Apache-LB, HAProxy or Varnish with the Change Environment Topology option.
Herewith, all the newly added nodes will be created at different hardware servers to ensure high-availability for your application.
Traffic Encryption with SSL
If your project requires some complex configurations for requests handling, like HTTPS and load balancing, feel free to leverage the following security options:
- built-in BitssCloud SSL functionality allows to instantly apply traffic encryption for your application internal domain (i.e. https://{env_name}{platform_domain}) with BitssCloud wildcard SSL certificate
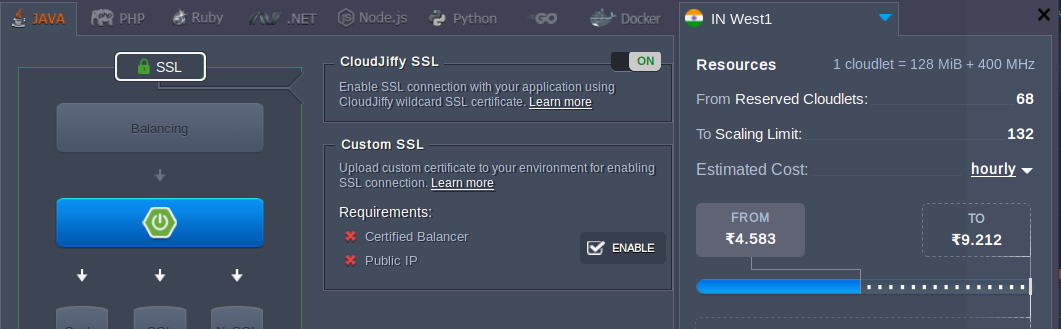
- as a free-of-charge SSL alternative, applicable for both internal and custom domains, a special BitssCloud-developed Let’s Encrypt add-on can be used
Applying any of these solutions to the Load Balancer node will enable traffic encryption on environment level, eliminating the necessity to configure it inside your application. As a result, the received encrypted traffic will be terminated at the load balancing layer and proxied further to the application server in a plain view.
Custom Ports & HTTP/HTTPS Usage Considerations
By default, the majority of Java applications listen to port 8080 on HTTP level, thus it is considered as the standard Spring Boot endpoint for HTTP traffic.
Herewith, when your environment link is requested over the Internet, the ports are automatically mapped as follows:
- internal HTTP port 8080 refers to the port 80
- secure HTTPS port 8043 refers to the ports 443 and 80
Thus, if working over standard HTTP/HTTPS ports, your application can be accessed directly via environment URL with the corresponding protocol specified, with no necessity to enter the appropriate port number.
And if your application does need to process the requests on some custom interface, BitssCloud allows exposing private container TCP and UDP ports via Endpoints. After adding such, the corresponding port will be automatically enabled in server firewall settings, making it accessible for the rest of the world.
Signup Now for a 14-day free trial to try out Springboot on BitssCloud.